How to I use Implement Digest Authentication in Node.js
App Development
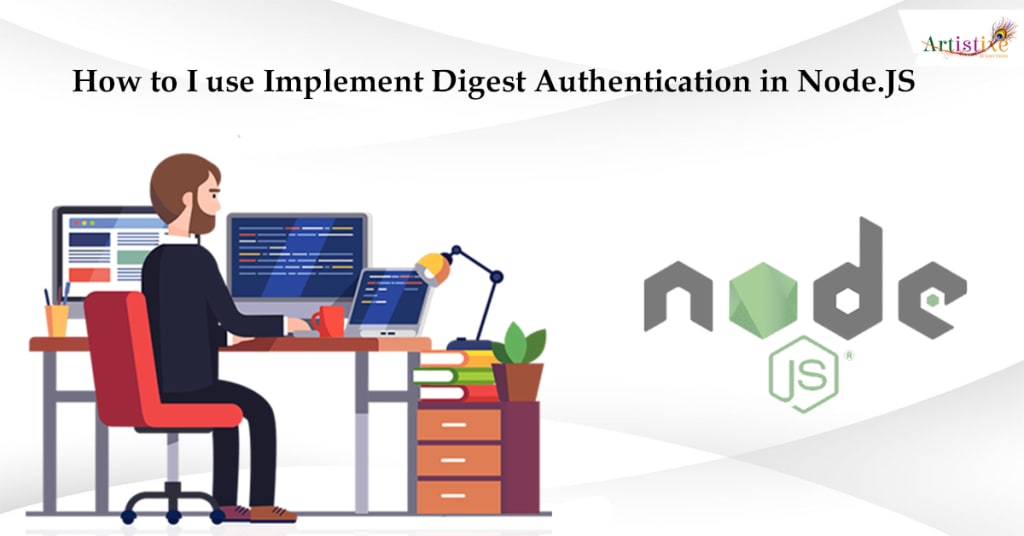
The Node.js framework is used for the back-end of web applications. It can handle concurrent requests and is scalable. It combines a runtime environment, JavaScript library, and encourages scaling-friendly design decisions. Compared to Erlang or Scala, it is easy to learn and use. A large number of great libraries are available, and it has an active open-source community. Toten untrusted client input builds simple to complex Node.js applications, one needs to find and install open-source dependencies, build out the rough directory structure by adding models, tests, assets, etc., and write code to link them together.
What to Consider Before Developing a Node.js App?
Consider these essentials about Node.js before moving forward:
- Node.js application structure
- Finding and installing interesting dependencies with npm
- Unit testing and better function decomposition will help you avoid spaghetti code
- Deploying your completed project
- Using npm to share your completed project
Install Node.js (nodejs.org/en/) in your favourite text editor and get ready to follow along. The application backend works beautifully with Node.js. Let's start with the features and finish with security best practices.
Features of Node.js
- Chrome's JavaScript Engine (V8 Engine) is the foundation for Node.js. It makes it run faster.
- An open-source runtime environment for the development of server-side and networking applications
- JavaScript is used by Node.js applications
- Uses the Node.js runtime on OS X, Windows, and Linux
- REPL Terminal
- Package Manager (NPM)
- Callbacks
- Event Loop
- Event Emitter
- Buffers
- Streams
- File Systems
- Global Objects
- Utility Modules
- Web Modules
- Express Framework
- RESRFul API
- Scaling Application
- Packaging
Each API is asynchronous, event-driven, and non-blocking. The Node.js server never waits for APIs to return data. After calling the API, the server moves to the next API, and Nide.js' Events mechanism enables it to receive a response from the previous API call.
Node.js processes data in chunks. It never buffers data.
It is used by companies such as eBay, GE, Microsoft, PayPal, Uber, Wikipins, Yahoo!, and Yammer.
What is the Purpose of Node.js?
Applications using Node.js include I/O based applications, Data Streaming Applications, JSON APIs based applications, and Single page applications. The single-threaded nature makes it an ideal server for non-blocking, event-driven applications. In traditional websites and back-end API services, they are built with a push-based, real-time architecture.
Security Best Practices For Node.js Applications
Make use of parameters to prevent injection attacks: Node.js applications are vulnerable to SQL injection attacks. The hacker intrudes on the system by sending queries as user inputs, which trigger the system to release sensitive information without the user's consent. It is never a good practice to pass parameters from the frontend to the database query without verifying them. Some Node.js libraries do this automatically. It is impossible to secure a Node.js application without validating the input from the user. Cross-site scripting/XSS attacks pose the same threat.
Use Multi-Factor Authentication to Prevent Automated Attacks: A broken authentication system will break any system. It may be a weak password, a weak session policy, or even a weak management policy. A Node.js application can implement two-factor authentication (2FA) using an npm package and generate one-time tokens using authentication service providers.
Discard Sensitive Data after Use: There is always a risk of exposing sensitive data. Attackers are always on the lookout for ways to steal secrets, hijack sessions, steal users' data, and perform man-in-the-middle attacks. Your passwords should be strong and encrypted with strong hashing functions. Implement HTTP strict transport security (HSTS) on TLS to prevent packet sniffing and man-in-the-middle attacks.
Patch Old XML Processors: XML external entities attacks allow XML processors to trick them into allowing data from external or local resources. It is possible to evaluate the specifications of external entities during the XML processing in older XML processors.
Enforce Access Control on Every Request: It's impossible from the client-side to manipulate access permissions through cookies or JWT (JSON Web Token) authorization tokens due to loosely-coupled control policies, insufficient functional testing, weak access control rules, and intrusion of middlewares. Set up log access controls and limit the API rate.
Create Fluid Build Pipelines for Security Patches: As a development, staging has loose patches due to security misconfigurations in soft build pipelines, node.js apps are vulnerable. Keep all environments identical with different credentials and distinct access levels. The default package settings, on the other hand, leave breadcrumbs for malicious attackers to follow.
Sanitize All Incoming Inputs: Hackers execute malicious JavaScript Code on client-side applications in cross-site scripting (XSS) attacks. This can be either client-side or server-side. Basically, it occurs when a client input that is untrusted is accepted by the backend Nginxut processing and storing. It may contain malicious JavaScript code.
Scan Application for Vulnerabilities Regularly: Many open-source vulnerabilities exist in node.js packages. Package manager-provided solutions and WhiteSource bolt let developers track them.
Secure Deserialization: The insecure deserialization flaw allows malicious objects to be executed via API calls or remotely. An object/data structure attack occurs when an attacker executes remote code on the application to modify it. An example is when legitimate data objects like cookies are tempered by a hacker for malicious purposes.
Cross-Site Request Forgery (CSRF): Create a CSRF token from the server and add it to a hidden form field to prevent such attacks.
Sufficient Logging and Monitoring: The attackers often intrude with all the tools in stock before the point of entry. As developers configure applications and services to a centralized logging system, they will be able to keep a record of ongoing attacks or attempted data breaches. Pentest exercises should be conducted regularly to resist logging and monitoring policies, and to assist teams in developing response and recovery plans if a breach occurs.
Eradicating Brute Force Attacks: Hackers use this technique to unlock applications by constantly generating random passwords. Use bcrypt.js as part of Node.js security to protect passwords stored in the database.
Security Against Denial-of-Service Attacks: The premature termination of processes is caused by exploiting bugs in HTTP handling. Utilize a limiting function that limits the number of requests a user can make by implementing a high limiting factor. By limiting the body payload, the number of data sent in the body payload is limited. It can be seen that the load is never higher than it needs to be.
Additionally,
Make use of security-related linter plugins to identify security vulnerabilities.
Middleware can be used to limit concurrent requests. You can limit concurrent requests with middleware such as a cloud load balancer, cloud firewall, Nginx, rate-limiter-flexible package or a rate-limiting middleware.
Use packages to encrypt configuration files: Even for private repositories, avoid making source control public.
Using ORM/ODM libraries to prevent query injection vulnerabilities: If you use JavaScript template strings or string concatenation to inject values into queries, you can open yourself up to a wide range of vulnerabilities. Data access libraries in Node.js are all protected against injection attacks.
Avoid DOS attacks: Errors cause the node process to crash. When a process crashes due to an unhandled error, raise an alert with critical severity. Make sure to validate all user input and not crash the process when invalid input is entered. Wrap all routes in catch-alls and consider not to crash when an error occurs within a request.
Adjust the HTTP response headers for enhanced security: Using common attacks like cross-site scripting (XSS), clickjacking, and other malicious attacks in the application form, utilize secure headers.
Continually and automatically inspect for vulnerable dependencies: Developers of Node.js use npm, audit, nsp, and snyk to monitor, track, and patch vulnerable dependencies. Detect your vulnerable dependencies before they are released with these tools integrated into your CI setup.
Exclude HTML, JS, and CSS output: Avoid cross-site scripting (XSS) by using dedicated libraries that mark data as pure content that will never be executed.
Validate incoming JSON schemas: Ensure that the incoming payload meets expectations. By using JSON-based validation schemas, you avoid tedious validation coding within each route.
Support blacklisting JWT tokens: If you are using JWT tokens, there is no way to revoke access if a token is issued. Implement a blacklist of untrusted tokens that will be validated upon request.
Run Node.js as a non-root user: By using the flag "u username", the container can be invoked on behalf of the non-root user by baking the non-root user into the Docker image.
Avoid JavaScript eval statements: JavaScript code can be evaluated during run time with eval. As well as improving performance, it also deals with security concerns caused by malicious JavaScript code originating from user input. Don't pass JavaScript code along with new functions, settimeouts, and setintervals.
Prevent evil RegEx from overloading your single thread execution: If you must write your Regex patterns, use a third-party package like validator.js instead, or use safe-regex to identify vulnerable regex patterns.
Avoid module loading using a variable: If the path to the file was given as a parameter, do not import it since it may have originated from user input. For early detection of such patterns, use the Eslint-plugin-security linter.
Run unsafe code in a sandbox: Isolate and safeguard the main code using a sandbox execution environment. Use a dedicated serverless process (cluster.fork()) or dedicated npm packages that act as a sandbox.
Take extra care when working with child processes: Shell-injection can be mitigated by using child_process.execFile, which runs a single command and does not allow shell parameter expansion.
Hide error details from clients:
Secure sensitive application information by hiding error details from the client –
(1) File paths on the server
(2) Use of third-party modules
(3) Internal workflows of the application that could be exploited by an attacker.
Modify session middleware settings: Use default settings for session middlewares to protect the application from the module and framework-specific hijacking attacks. Hide identifiers and details about your technology stack.
Do not publish secrets to the npm registry: Take necessary precautions to avoid accidentally exposing secrets to public npm registries. Use the .npmignore file to blacklist specific files or folders, or package.json as a whitelist;
Conclusive: We can help you with Node.js App Development
In addition to security, the need to stick to a strict deadline sometimes prevents Node.js developers from properly implementing security measures in their Node.js Applications. Throughout the software development life cycle, from conception to production, we configure and cross-check security. We hope you now have a better understanding of node.js security issues and node.js security best practices. Contact us to learn more!
About the Creator
Artistixe IT Solutions LLP
With top-notch & affordable solutions, Artistixe IT Solution is a leading mobile and web development company in India which is focusing on iOS and Android applications development.
Comments
There are no comments for this story
Be the first to respond and start the conversation.