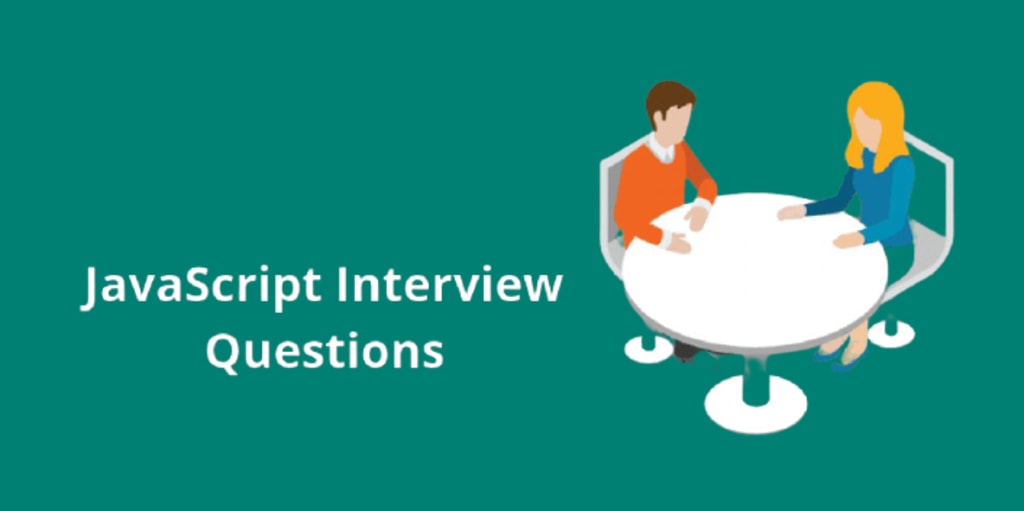
1. Difference between “ == “ and “ === “ operators.
Both are comparison operators. The difference between both the operators is that “==” is used to compare values whereas, “ === “ is used to compare both values and types.
Example:
var x = 100;
var y = "100";
(x == y) // Returns true since the value of both x and y is the same
(x === y) // Returns false since the typeof x is "number" and typeof y is "string"
2. What is NaN property in JavaScript?
NaN property represents the “Not-a-Number” value. It indicates a value that is not a legal number.
typeof of NaN will return a Number.
To check if a value is NaN, we use the isNaN() function
Note- isNaN() function converts the given value to a Number type, and then equates to NaN.
isNaN("Nishar") // Returns true
isNaN(1928) // Returns false
isNaN('1') // Returns false, since '1' is converted to Number type which results in 0 ( a number)
isNaN(true) // Returns false, since true converted to Number type results in 1 ( a number)
isNaN(false) // Returns false
isNaN(undefined) // Returns true
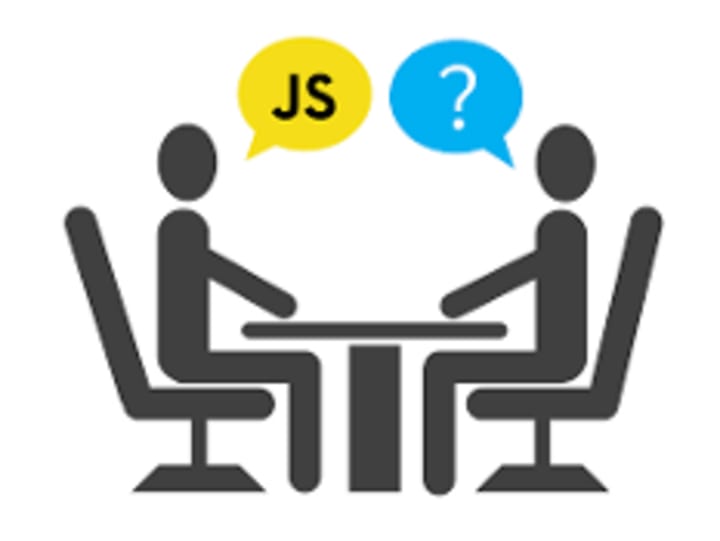
3. What are the different data types present in javascript?
To know the type of a JavaScript variable, we can use the typeof operator.
1. Primitive types.
String — It represents a series of characters and is written with quotes. A string can be represented using a single or a double quote.
Example :
var name = "Nishar Multani"; //using double quotes
var str2 = 'multani nishar'; //using single quotes
Number — It represents a number and can be written with or without decimals.
Example :
var x = 35; //without decimal
var y = 34.6; //with decimal
BigInt — This data type is used to store numbers which are above the limitation of the Number data type. It can store large integers and is represented by adding “n” to an integer literal.
Example :
var bigInteger = 234567890123456789012345678901234567890;
Boolean — It represents a logical entity and can have only two values : true or false. Booleans are generally used for conditional testing.
Example :
var a = 2;
var b = 3;
var c = 2;
(a == b) // returns false
(a == c) //returns true
Undefined — When a variable is declared but not assigned, it has the value of undefined and it’s type is also undefined.
Example :
var x; // value of x is undefined
var y = undefined; // we can also set the value of a variable as undefined
Null — It represents a non-existent or a invalid value.
Example :
var z = null;
Symbol — It is a new data type introduced in the ES6 version of javascript. It is used to store an anonymous and unique value.
Example :
var symbol1 = Symbol('symbol');
typeof of primitive types :
typeof "John Doe" // Returns "string"
typeof 3.14 // Returns "number"
typeof true // Returns "boolean"
typeof 234567890123456789012345678901234567890n // Returns bigint
typeof undefined // Returns "undefined"
typeof null // Returns "object" (kind of a bug in JavaScript)
typeof Symbol('symbol') // Returns Symbol
2. Non-primitive types.
Primitive data types can store only a single value. To store multiple and complex values, non-primitive data types are used.
Object — Used to store collection of data.
Example:
// Collection of data in key-value pairs
var obj1 = {
x: 43,
y: "Hello world!",
z: function(){
return this.x;
}
}
// Collection of data as an ordered list
var array1 = [5, "Hello", true, 4.1];
Note- It is important to remember that any data type that is not a primitive data type, is of Object type in javascript.
4. Explain passed by value and passed by reference.
In JavaScript, primitive data types are passed by value and non-primitive data types are passed by reference.
For understanding passed by value and passed by reference, we need to understand what happens when we create a variable and assign a value to it,
Example:
var x = 2;
In the above example, we created a variable x and assigned it a value of “2”. In the background, the “=” (assign operator) allocates some space in the memory, stores the value “2” and returns the location of the allocated memory space. Therefore, the variable x in the above code points to the location of the memory space instead of pointing to the value 2 directly.
Assign operator behaves differently when dealing with primitive and non-primitive data types,
Assign operator dealing with primitive types:
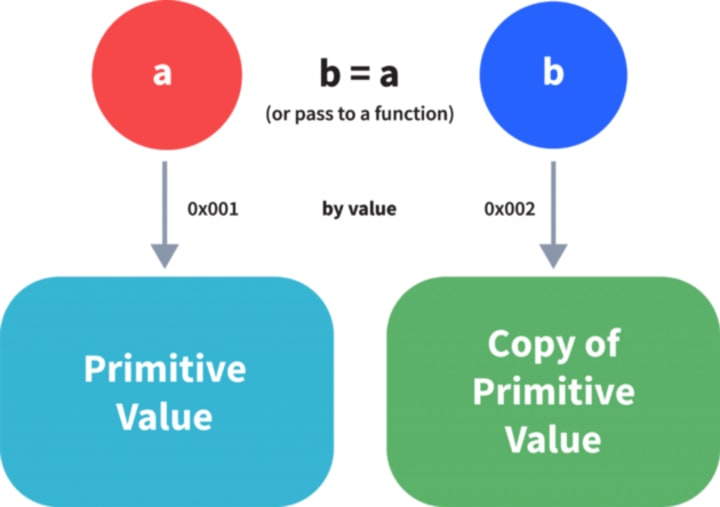
Example:
var y = 234;
var z = y;
In the above example, the assign operator knows that the value assigned to y is a primitive type (number type in this case), so when the second line code executes, where the value of y is assigned to z, the assign operator takes the value of y (234) and allocates a new space in the memory and returns the address. Therefore, variable z is not pointing to the location of variable y, instead, it is pointing to a new location in the memory.
Assign operator dealing with non-primitive types:
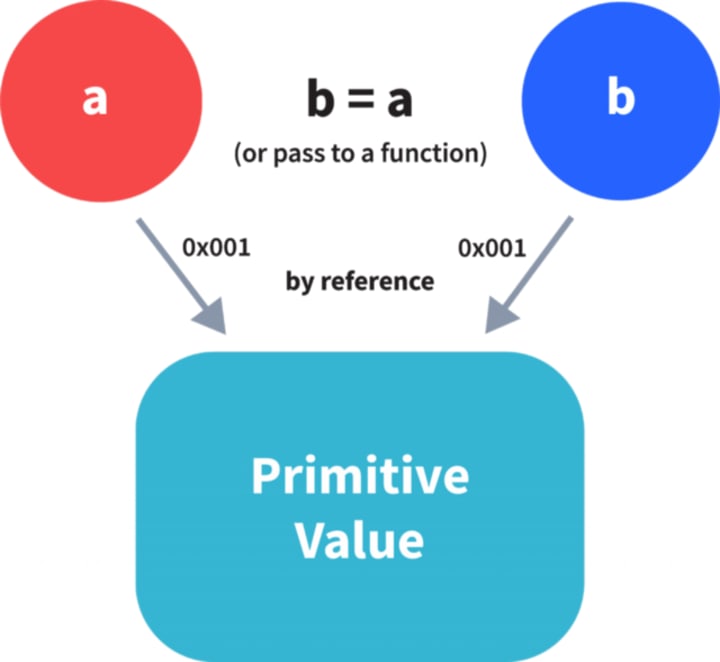
var obj = { name: "Nishar", lastName: "Multani" };
var obj2 = obj;
In the above example, the assign operator directly passes the location of the variable obj to the variable obj2. In other words, the reference of the variable obj is passed to the variable obj2.
var obj = #8711; // obj pointing to address of { name: "Nishar", lastName: "Multani" }
var obj2 = obj;
var obj2 = #8711; // obj2 pointing to the same address
// changing the value of obj1
obj1.name = "Zaid";
console.log(obj2);
// Returns {name:"Zaid", lastName:"Multani"} since both the variables are pointing to the same address.
From the above example, we can see that while passing non-primitive data types, the assign operator directly passes the address (reference).
Therefore, non-primitive data types are always passed by reference.
5. Difference between var and let keyword in javascript.
Some differences are
- From the very beginning, the ‘var’ keyword was used in JavaScript programming whereas the keyword ‘let’ was just added in 2015.
- The keyword ‘Var’ has a function scope. Anywhere in the function, the variable specified using var is accessible but in ‘let’ the scope of a variable declared with the ‘let’ keyword is limited to the block in which it is declared. Let’s start with a Block Scope.
- In ECMAScript 2015, let and const are hoisted but not initialized. Referencing the variable in the block before the variable declaration results in a ReferenceError because the variable is in a “temporal dead zone” from the start of the block until the declaration is processed.
About the Creator
lover
I am a full stack developer. in my free time i like to research in this huge network and find something unique. and share
Comments (1)
Its good.