How to Implement a 'Git Submodule Library' in an Android Project? (2024)
Learn how to create and integrate a Git Submodule library into your Android projects for efficient code management.
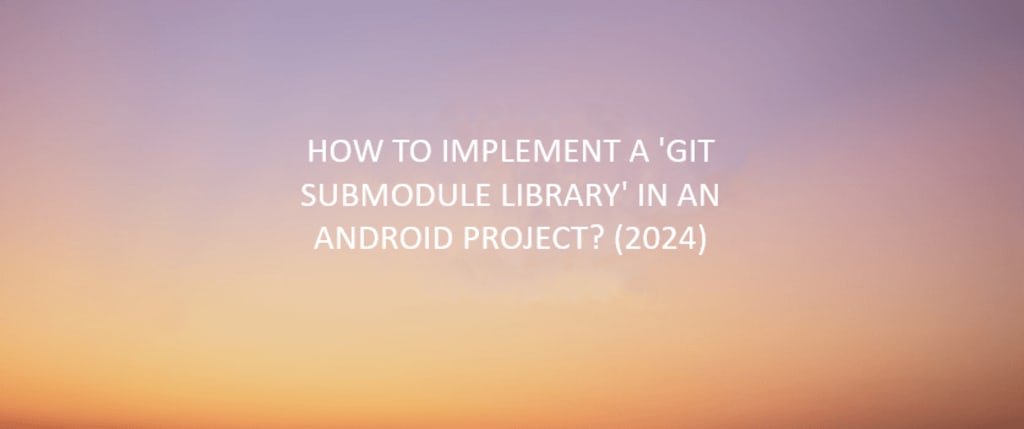
In this article, I'll answer the question of "How to create a Git Submodule library?" but I'm not sure exactly where to start. The best place to begin is probably to go back to the time when I first asked myself, "How do you add one Android project to another?" Why did such a need arise and when?
Everything started in the spring months of 2019. During my technical interview process at Peakup, while the hiring process was ongoing, I bombarded Peakup Labs department manager Emrah Uslu with many questions such as "How many projects are we going to do?", "How do you plan to make the projects?", "Do we have a Native Mobile developer on the team? (This question implies the presence of Legacy code and needs to be asked)" … etc. He replied to me, "There are plenty of projects to be done, don't worry," and added that instead of a single large project lasting for years, we would be working on many small to medium-sized projects.
The idea of a "library that can be used across different projects" that had occurred to me at my previous job seemed sensible once again. I don't remember if I mentioned this to my manager during the interview, but from my first day at work, he specifically requested me to create such a shared library.
For instance, every project of ours has a "Login page" and it's identical in all of them. Since the company was a Microsoft partner, we'll be adding Microsoft's login page as a WebView. Alongside common pages like language selection, theme settings, we'll be writing dozens of methods, customized styles and themes, various custom views that will form the common design language, extension functions, web service request architecture, DSL… Phew! It all needs to be present in every project, and to top it off, they're all the same. Rather than falling into the copy-paste loop and turning projects into trash, isn't it simpler and more reasonable to add these common pages and features to a separate library and use them collectively from there?
I've experienced firsthand the huge downside of copy-pasting before: There were 8–10 projects created by forking each other. The slightest service change, bugfix, refactor… meant making the same change over and over again like a laborer across all these projects. No matter how you spin it, this isn't a good example of development. Don't do it. Stay away. You can utilize the fork feature much more efficiently, so use it.
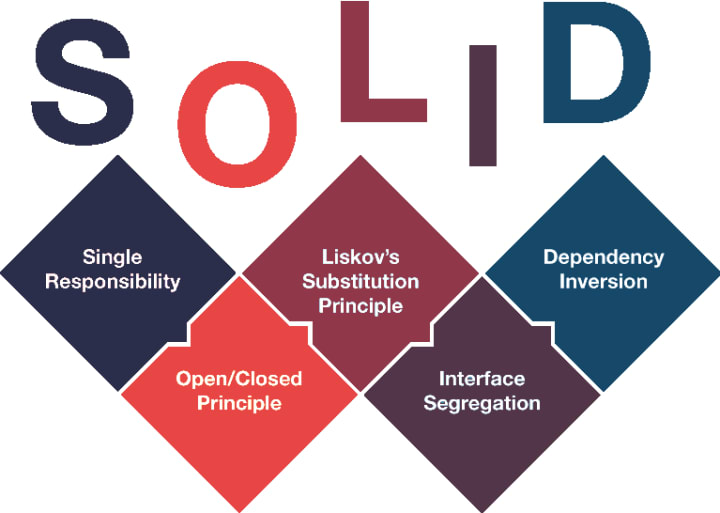
When you think about it, you'll see that the Open/Closed Principle, Software Reuse (Don't Repeat Yourself), and even the SOLID principles support library systems; they don't endorse copy-paste coding as a healthy practice.
Technically Speaking?
I reckon I've done a decent job explaining what the "Git Submodule Project" is and why it's needed. If you're thinking, "Okay, got it, but how are you gonna pull this off technically?" then here's the scoop:
When I first heard "library," my mind jumped straight to JAR files. But turns out, JAR files can only host classes from the Java SDK; they can't handle classes from the Android SDK like Activity, Fragment, SharedPreferences, WifiManager… And guess what? My library needs classes like Activity and SharedPreferences. So, another type of library that comes to mind is AAR files.
Now, AAR files have a few drawbacks: One of the biggest pains is that every time you add any code, you gotta create a new AAR file and add it to your main project. When you're at a place like Peakup where there's no legacy code lying around and both the library and the main apps are gonna be written from scratch, side by side, dealing with AAR files can be a real headache. Sure, if the library was already written and prepped, I'd go for AAR since it takes up less space.
Here are the must-have features for our dream library:
Flexibility, Adaptability to constant change (It shouldn't give developers a headache during these changes), Compatibility with the Open/Closed Principle to an almost religious degree, and working straight out of VSTS (Git).
Once we identified the needs, the choice became crystal clear. Enter Git's shining star: Submodule.
The library had to include a standard Authenticator layer that would be used consistently across all projects. You know, the good ol' login screen. Since this would be the library's main gig, I named it PeakAuth; it became like my baby. I loved it, nurtured it, kept adding to it until I switched over to iOS. My first Android app at Peakup was EnviSense, and I kept thinking, "Oh, I can use this extension function in other projects too! Let me add it to PeakAuth. Oh, why should I rewrite these date formats in every project? Let's add them to PeakAuth right away…" And just like that, bit by bit, piece by piece, the library grew with shared features and code snippets. I mentioned it was like a child, right? Well, it was exactly like that - my little bundle of joy.
Note: Since Android Git Submodule is a feature of Git, our main project must also reside within Git in the library. I won't even delve into the importance and reasons for using Git. Also, don't worry about the project ID parts in the screenshots; I didn't have access to my previous company's project, so I had to recreate them in a new project.
How to Turn an Application into an Android Git Submodule?
We've opened our project in Android Studio, where we'll later turn it into a library, and we've written our shared code. Now it's time to transform it into a library.
Here are the 3 simple steps we need to take:
In Android, a library project cannot have a launcher activity. No activity in the AndroidManifest file can have the Launcher tag. You can simply turn it into a comment:
<activity android:name=".MainActivity" android:exported="true">
<!-- <intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>-->
</activity>
In Android, a library project, in the app level gradle.build file, boasts the com.android.library tag at the top, not the com.android.application tag like regular apps.
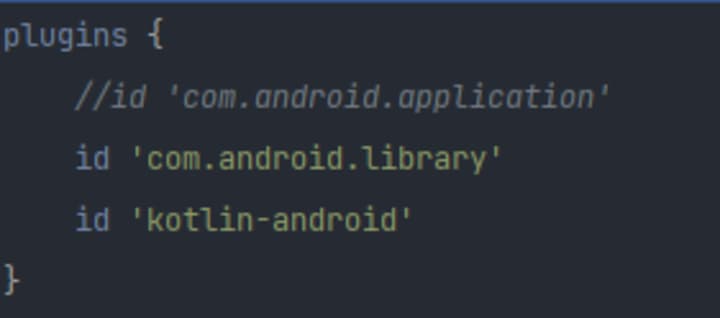
In the same file as point 2, under the default config section, the applicationId, typically found in applications, should not exist in libraries; it should be deleted.
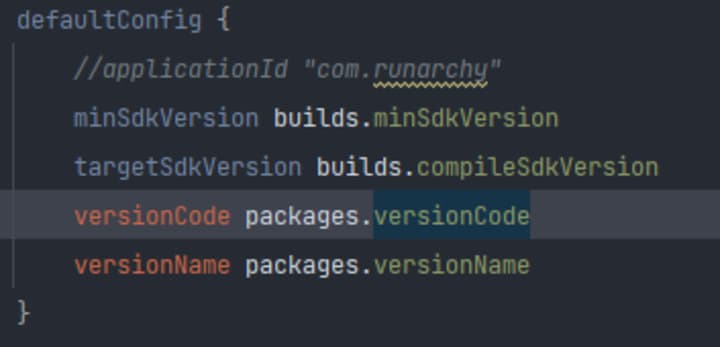
Sync it, commit & push it, and voila! PeakAuth has now transformed into a library that can be shared across other projects.
How to Add a Git Submodule Library to Another Android Project?
After creating our library project, it's time to dive into how we'll use it and add it to Android projects. You might want to jot this down in the README file on Git for ease of access, especially if you're working in a team. Important points like these shouldn't rely on just one developer in the company. It should be easily understood, added, and used not only by the creator but also by other developers.
I believe I need to emphasize a crucial point right from the start: Syncing should not be done until the last step I mention below. Syncing will only be done after all connections and settings are completed.
To add the library to your project, open the project in the terminal using the cd command. Then, write the code shown below and add the link to the library:
git submodule add https:…
Or, you can add it more simply via SourceTree. If you're adding a Git Submodule to your project - especially if it has a dynamic purpose like mine - I suggest using SourceTree. Managing the Submodule within the project will be easier. When you open the project in SourceTree, right-click in the empty space on the left, as shown in the screenshot below, and select Add Submodule.
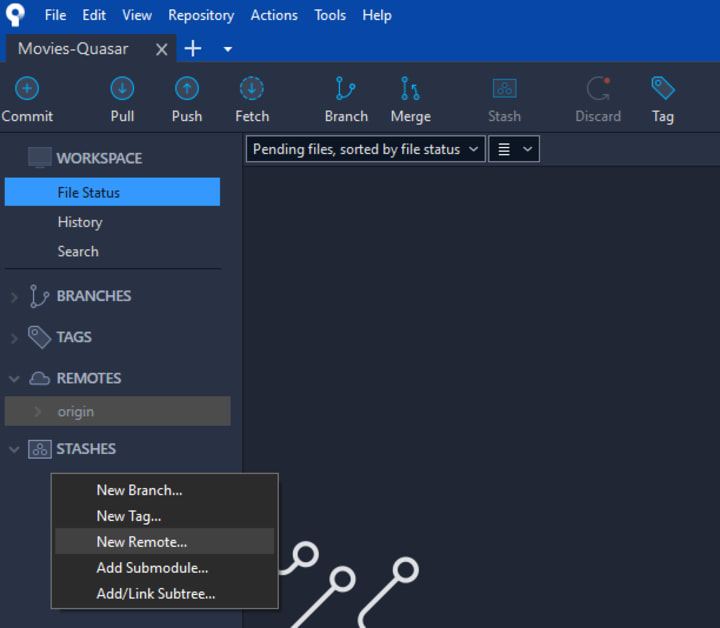
In the window that pops up, enter the necessary information and the link to the Submodule. If the Submodule has multiple branches, you can use the Advanced Options to specify which branch you want to link to. Then, of course, you need to hit the OK button. The name you give to the file you're adding here is crucial. As Peakup Labs mobile developers, we naturally name the file where the PeakAuth library will reside "peakauth."
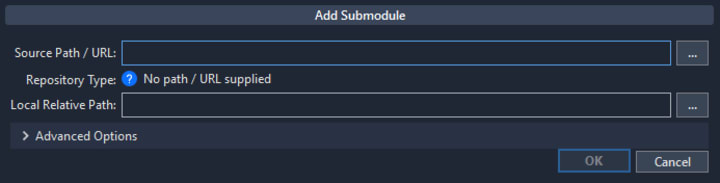
After completing this step or when you run the git submodule add command, the .gitmodule file will be automatically added to your main project.
Navigate to the settings.gradle file in your project and add the following lines:
:include ':peakauth'
project(':peakauth').projectDir = new File('peakauth/app')
In the application level build.gradle file of the project, add the following line as a dependency:
implementation project(":peakauth")
Sync it up. Like I mentioned earlier, syncing should not be done until this step.
If you accidentally synced at any intermediate step, you'll need to reset/discard all changes via Git, delete all added files, and start the process from scratch. Go back to the last commit. (You can also run "Git reset HEAD") I've done it a few times myself, and couldn't find a cleaner way than starting over. Also, I recommend committing before starting the library adding process. No other changes should be made so that you don't lose any code when doing a bulk reset.
If your project looks as snuggly nested, congrats, your Android Submodule library is ready to rock and roll! As you can see, you can frolic around in it to your heart's content, a feature that's not typically found in AAR-type libraries. Also, that snazzy icon at the top? It's a dead giveaway that peakauth is indeed a library - pretty cool, right? Plus, in the screenshot below, you get a sneak peek of how your project should strut its stuff in SourceTree.
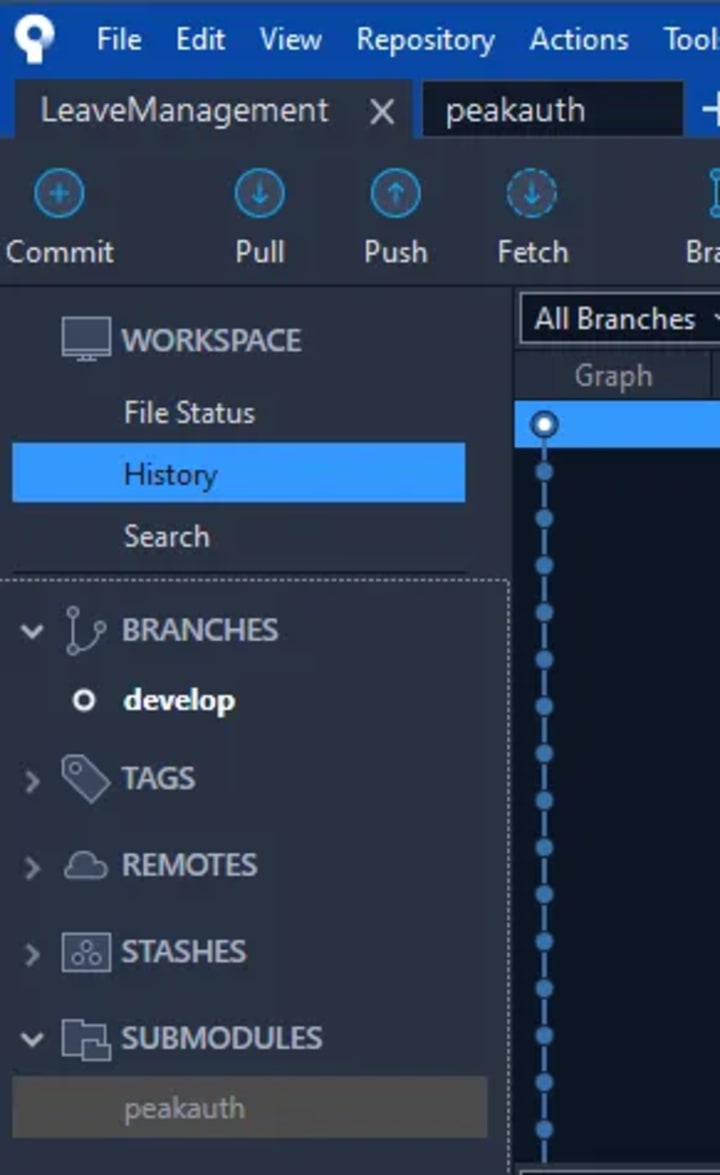
When you double-tap on the 'peakauth' text below, don't freak out if nothing happens - it's just that the peakauth submodule file in the project is currently playing hide and seek (a.k.a., it's empty). To get the ball rolling, you might need to dive into the terminal, strut your stuff with the 'cd' command to open the submodule's file, and then do a little magic trick called 'git clone' to fetch the submodule project. Once you've aced this step, voilà! Clicking on it will whisk you away to a new tab where our submodule project is ready to take the stage.
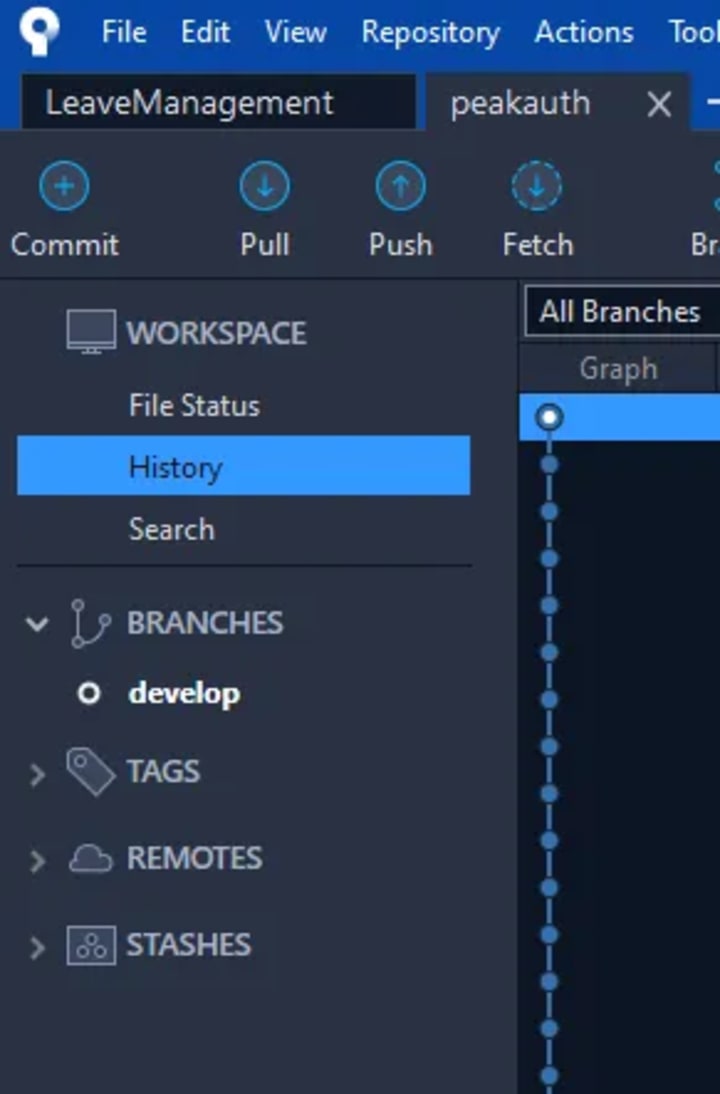
Life-Saving Note: Update the submodule only from its own Repository, from within itself. If we update PeakAuth inside EnviSense, it might ripple out to the real PeakAuth and throw a wrench in the works for other projects using the library. If you're not careful with the submodule, you might stir up a real hornet's nest. As cute and cuddly as its management might seem, it can get trickier as projects grow.
If you're hungry for more knowledge about using Submodule libraries in Android, the two sources below were lifesavers for me. I'm tucking them in here for you:
https://medium.com/@deepakpk/how-to-add-a-git-android-library-project-as-a-sub-module-c713a653ab1f
https://proandroiddev.com/creating-a-library-for-android-ea976983db1
This article originally made its grand debut on the blog of Peakup, a company I had the pleasure of working with. But since the screenshots got all wonky over there and it wasn't crystal clear that I'm the mastermind behind the words, I decided to teleport it over here. I also wrote this article in Medium
Wishing you days filled with kindness, love, and heaps of good reads!
About the Creator
Alparslan Selçuk Develioğlu
8+ years experienced Android Dev. Freshly a Software Team Leader. Colorful, confident personality, a fan of science fiction and fantasy works. An Ultratrail runner who runs in races 60+ kms
Enjoyed the story? Support the Creator.
Subscribe for free to receive all their stories in your feed. You could also pledge your support or give them a one-off tip, letting them know you appreciate their work.
Comments (1)
Thank you for the interesting and delicious content. Follow my story now.