Advanced API in Flutter: An In-Depth Guide
Understanding the Power and Flexibility of the Flutter Framework
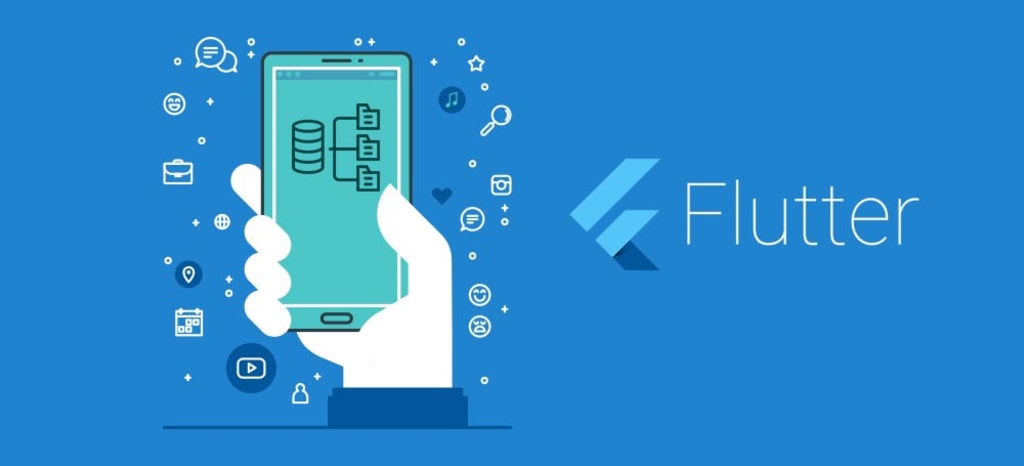
Flutter is an open-source framework that provides a modern and expressive way of building mobile applications. Its hot reload feature and a wide range of available libraries make it a popular choice among developers. One of the key components of Flutter is its advanced API, which provides the tools for developing high-quality applications with a focus on performance, ease of use, and extensibility. In this blog post, we'll explore the advanced API in Flutter, its features, and benefits, and provide code examples to illustrate its usage.
What is an API in Flutter?
An API, or Application Programming Interface, is a set of tools and protocols that allow two software applications to communicate with each other. In the case of Flutter, the API provides developers with a range of functionality that can be used to build their applications. It includes basic functionality, such as text rendering and layout, as well as more advanced features, such as animation and network connectivity.
What are the features of the Advanced API in Flutter?
The advanced API in Flutter offers a range of features that can be used to build high-quality, modern mobile applications. Some of the key features include:
1. Animations: The advanced API provides a range of tools for creating smooth and responsive animations, such as tweens and curves.
2. Network connectivity: Flutter provides libraries for making network requests and consuming web services, allowing developers to build connected applications.
3. Routing: The advanced API provides a robust routing system, making it easy to navigate between different screens and pages within an application.
4. Text rendering: Flutter provides a range of text-rendering tools, including rich text, Unicode support, and font customization.
5. Widgets: Flutter includes a range of widgets that can be used to build the user interface of an application, including buttons, sliders, and lists.
Benefits of the Advanced API in Flutter
• Improved performance: The advanced API in Flutter is optimized for performance, making it a great choice for applications that need to be fast and responsive.
• Easy to use: The API is designed to be easy to use, with clear and concise documentation, making it accessible to developers of all skill levels.
• Extensibility: The advanced API is designed to be highly extensible, allowing developers to add their own functionality and libraries to the framework.
Code Examples
Here are some code examples that illustrate the usage of the advanced API in Flutter:
1. Animations:
To create an animation in Flutter, you can use the AnimationController class, which provides control over the animation timeline. Here is an example of a simple animation that scales an image from its original size to twice its size:
class ScaleAnimation extends StatefulWidget {
@override
_ScaleAnimationState createState() => _ScaleAnimationState();
}
class _ScaleAnimationState extends State<ScaleAnimation>
with SingleTickerProviderStateMixin {
AnimationController controller;
Animation<double> scaleAnimation;
@override
void initState() {
super.initState();
controller = AnimationController(
vsync: this,
duration: Duration(seconds: 1),
);
scaleAnimation = Tween<double>(begin: 1, end: 2).animate(controller);
controller.forward();
}
@override
Widget build(BuildContext context) {
return Scaffold
(
body: Center(
child: AnimatedBuilder(
animation: controller,
builder: (BuildContext context, Widget child) {
return Transform.scale(
scale: scaleAnimation.value,
child: Image.asset('assets/image.png'),
);
},
),
),
);
}
},
2. Network connectivity:
To make a network request in Flutter, you can use the `http` package. Here is an example of a function that fetches data from a remote API:
Future<List<Photo>> fetchPhotos() async {
final response = await http.get('https://jsonplaceholder.typicode.com/photos');
if (response.statusCode == 200) {
final photos = json.decode(
response.body).cast<Map<String, dynamic>>();
return photos.map<Photo>((json) =>
Photo.fromJson(json)).toList();
}
else {
throw Exception('Failed to load photos');
}
}
3. Routing:
To navigate between different screens in Flutter, you can use the `Navigator` widget. Here is an example of a button that navigates to a new screen:
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: RaisedButton(
child: Text('Go to next screen'),
onPressed: () {
Navigator.push(
context, MaterialPageRoute(
builder: (context) => SecondScreen()
),
);
},
),
),
);
}
}
class SecondScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text('This is the second screen'),
),
);
}
}
4. Text rendering:
To render text in Flutter, you can use the `Text` widget. Here is an example of a simple text widget:
class MyText extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Text( 'Hello, World!',
style: TextStyle(fontSize: 24),
);
}
}
5. Widgets:
To build the user interface of an application in Flutter, you can use a variety of widgets, such as buttons, sliders, and lists. Here is an example of a simple list widget:
class MyList extends StatelessWidget {
final List<String> items = ['Item 1', 'Item 2', 'Item 3'];
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(items[index]),
);
},
);
}
}
Conclusion
The advanced API in Flutter provides a range of features and tools for building high-quality, modern mobile applications. With its focus on performance, ease of use, and flexibility, Flutter enables developers to create fast, beautiful, and responsive applications that run smoothly on both iOS and Android platforms. Whether you are looking to create animations, make network requests, navigate between screens, render text, or build complex user interfaces, the Flutter API has everything you need.
In conclusion, if you are looking to build modern, high-quality mobile applications, the Flutter framework is an excellent choice. With its advanced API, rich set of tools and widgets, and focus on performance and user experience, it provides a powerful platform for creating engaging, responsive applications that stand out from the crowd.
I hope this article has added to your knowledge and you enjoyed reading it. I request you to subscribe or follow me for more such blogs. Please mention your feedback in comments.
If you want me to write a blog on any topic of your wish, please mention that in comment.
You can find me on following social media platforms.
My Official Website:
www.thetecplanet.com
www.thetecmart.com
Follow Programming Hub on Facebook.
https://www.facebook.com/profile.php?id=100089858752142
Follow me on LinkedIn:
https://www.linkedin.com/in/mohammad-azeem-b37431161/
Follow me on Twitter:
https://twitter.com/Mohamme49054008
Cheers!
Comments
There are no comments for this story
Be the first to respond and start the conversation.