Java Exceptions: A Guide to Unlocking Their Potential
Java Exceptions
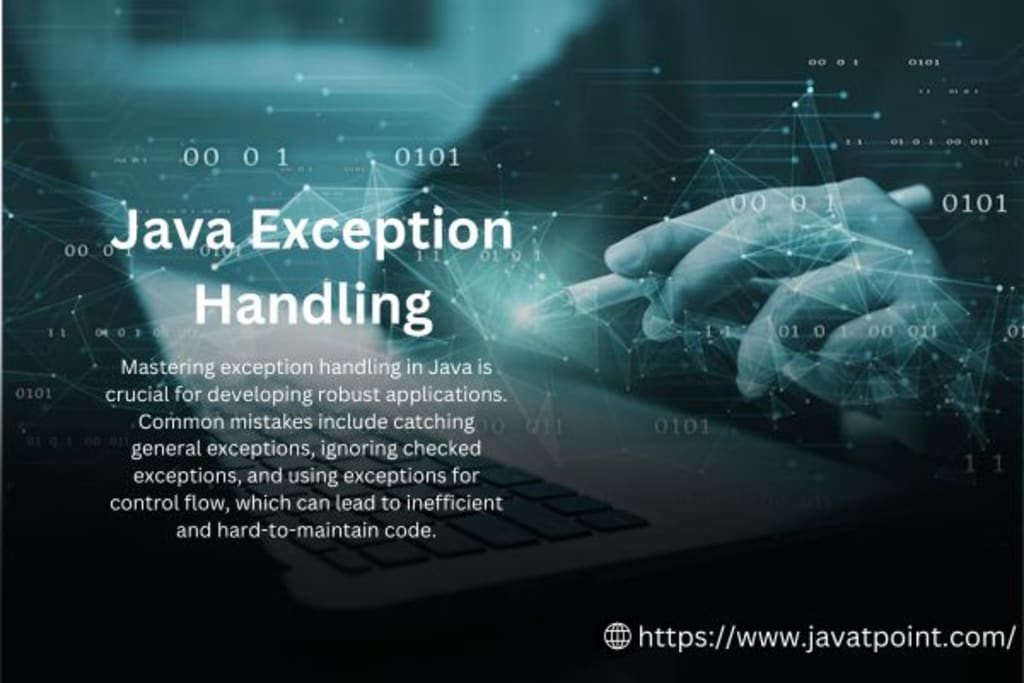
Java exceptions play a pivotal role in ensuring software reliability by handling unexpected errors gracefully. In Java, exceptions, categorized into checked and unchecked types, provide a mechanism to anticipate and manage unforeseen situations during program execution.
JAVATPOINT, a renowned resource for Java tutorials, offers comprehensive guides on how to effectively utilize Exceptions in Java programming.
Understanding these mechanisms not only improves code stability but also enhances debugging and maintenance processes, ultimately leading to more robust and user-friendly applications. Embracing Java exceptions empowers developers to unlock their full potential in creating resilient software solutions.
Understanding Java Exceptions
An exception in Java is an event that disrupts the normal flow of the program's execution. It is an object that the Java Virtual Machine (JVM) or the application code throws when an unexpected situation arises. Java exceptions are categorized into three main types:
1. Checked Exceptions: These exceptions are checked at compile-time. They are subclasses of `Exception` (excluding `RuntimeException`). Examples include `IOException` and `SQLException`. Handling checked exceptions is mandatory, ensuring that the programmer anticipates and manages potential problems.
2. Unchecked Exceptions: These exceptions are not checked at compile-time but at runtime. They are subclasses of `RuntimeException` and include exceptions like `NullPointerException` and `ArrayIndexOutOfBoundsException`. Unchecked exceptions typically indicate programming errors that could have been avoided with proper coding practices.
3. Errors: These are serious issues that a typical application should not try to handle. They are subclasses of `Error`, such as `OutOfMemoryError` and `StackOverflowError`, and generally indicate problems within the JVM or hardware.
The Importance of Exception Handling
Proper exception handling is crucial for several reasons:
- Maintains Application Stability: By catching and handling exceptions, applications can continue running smoothly even when unexpected events occur.
- Improves Debugging and Maintenance: Detailed exception handling can provide valuable information about what went wrong, making it easier to debug and maintain the code.
- Enhances User Experience: Informative error messages and graceful recovery from errors can significantly improve the user experience.
Best Practices for Effective Exception Handling
To unlock the full potential of Java exceptions, follow these best practices:
1. Use Specific Exceptions:
Catch specific exceptions rather than using generic catch blocks. This approach provides more detailed information about the error and allows for more precise handling.
try {
// Code that may throw an exception
} catch (FileNotFoundException e) {
// Handle specific exception
} catch (IOException e) {
// Handle other I/O exceptions
}
2. Avoid Swallowing Exceptions:
Do not catch exceptions without handling them or rethrowing them. Silent failures make debugging difficult and can lead to unexpected behavior.
try {
// Code that may throw an exception
} catch (Exception e) {
// Log or handle the exception appropriately
e.printStackTrace();
}
3. Use Finally Blocks:
Use `finally` blocks to ensure that resources are released, regardless of whether an exception was thrown. This is particularly useful for closing files, database connections, and other resources.
try {
// Code that may throw an exception
} catch (Exception e) {
// Handle the exception
} finally {
// Cleanup code
}
4. Custom Exceptions:
Create custom exception classes for specific error scenarios in your application. This can make your code more readable and maintainable.
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
5. Log Exceptions:
Always log exceptions using a logging framework. Logs are invaluable for diagnosing problems in production environments.
try {
// Code that may throw an exception
} catch (Exception e) {
Logger.getLogger(MyClass.class.getName()).log(Level.SEVERE, null, e);
}
Conclusion
Understanding Exceptions in Java is pivotal for writing stable and resilient applications. By adhering to best practices like using specific exception handling, avoiding swallowing exceptions, and employing `finally` blocks for resource management, developers can enhance code reliability and user experience. Resources like Javatpoint provide comprehensive guides and tutorials that deepen understanding of Java exceptions, making it easier to debug and maintain code. Embrace exceptions as valuable tools in programming, leveraging them to gracefully manage unexpected errors and ensure applications run smoothly in diverse environments.
About the Creator
Enjoyed the story? Support the Creator.
Subscribe for free to receive all their stories in your feed. You could also pledge your support or give them a one-off tip, letting them know you appreciate their work.
Comments (2)
Rahul, you did a great job.
Hey, just wanna let you know that this is more suitable to be posted in the 01 community 😊