What Are Some Java Programming Best Practices?
Java practices to make your code efficient
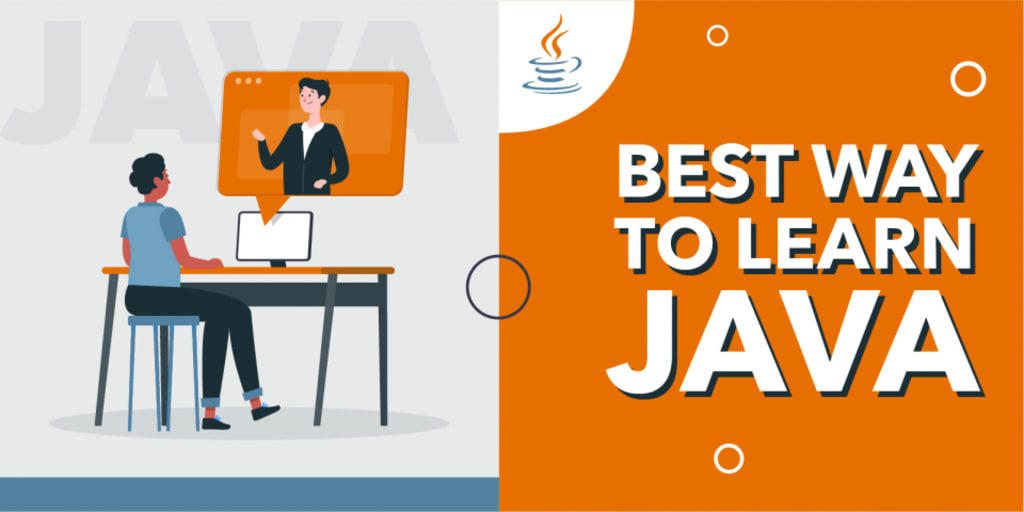
Java is one of the predominant programming languages. It is not only relevant today but quickly growing with the changing trends. According to Oracle, Java was the top programming language for hot applications such as Big Data, DevOps, Virtual reality, Artificial intelligence, Mobile, and micro services development.
Due to its relevance in the new technologies, it’s an essential skill to have. It is the best choice to start your programming career. You need to adopt the best standards. Follow to stay ahead of the competition. Follow the below Java practices to make your code efficient.
Java Programming Best Practices
Specify Naming Conventions
Setting a proper naming convention for your Java project is the best Java practice. You have to name every class, method, interface, variable, constant, etc. It will help maintain uniformity if you are working with other developers on the same projects. But you have to be careful while assigning names. The names should be meaningful, self-explanatory, readable, and easily identifiable by you and your teammates. You can use oracle Java code conventions as a reference for naming. Let’s take a look at some general naming rules:
• When naming class and interface, use nouns with the first letter in uppercase such as Painter, Rectangle, Student, Car, etc.
• For variable names, use nouns, beginning with a lowercase letter. For example, counter, number, gender, birthday, etc.
• Verbs should be used for method names, starting with a lowercase letter. For example: start, run, execute, stop, etc.
• Use uppercase for naming constant with words separated by underscores. For example: MIN_WIDTH, MAX_SIZE, MIN_HEIGHT, etc.
• For names, use camel Case notation such as Student Manager, number Of Students, Car Controller, and run Analysis, etc.
Ordering Class Members By Scope Of Accessibility
Minimize the accessibility of class members(field) and keep it as inaccessible as possible. A private access modifier is ideal for protecting the fields. This practice is recommended for maintaining encapsulation, a fundamental concept of OOP. It’s a basic concept in object-oriented programming, and many developers are aware of it. But most of them don’t know how to appropriately assign access modifiers to the classes and keep them public. Look at the following class whose fields are made public.
public class Student {
public String name;
public int age;
}
This is a sign of poor design as anyone can change this program. Now, look at a better design.
public class Student {
private String name;
private int age;
public void setName(String name) {
if (name == null || name.equals("")) {
throw new IllegalArgumentException("Name is invalid");
}
this.name = name;
}
public void setAge(int age) {
if (age < 1 || age > 100) {
throw new IllegalArgumentException("Age is invalid");
}
this.age = age;
}
Use Underscores In Long Numeric Literals
The next feature is an update introduced in Java 7. It makes lengthy numeric literals readable. So, consider this old way of writing:
- int maxUploadSize = 20971520;
- long accountBalance = 1000000000000L;
- float pi = 3.141592653589F;
Instead write it in a readable way like this:
- int maxUploadSize = 20_971_520;
- long accountBalance = 1_000_000_000_000L;
- float pi = 3.141_592_653_589F;
Avoid Leaving Empty Catch Bugs
Not leaving the empty catch blogs is the best online Java practice preferred by experienced developers. This is because when the empty catch block finds an exception, the program fails without showing anything. It makes debugging difficult and time-consuming.
Use StringBuilder or StringBuffer :
It’s a common practice to join the strings with the “+” operator in many programming languages, including Java. But it proves inefficient when you need to concatenate numerous strings as the Java compiler creates the final concatenated strings only after creating multiple intermediate String objects. In this case, “StringBuilder” or “StringBuffer” is ideal instead of String Concatenation as it saves a lot of processing time and unnecessary memory usage.
Avoid Initializing The Variables Explicitly
Even though it’s a widespread practice, you should not initialize member variables with values such as 0, false, and null as they already are default initialization values of member variables. So, the best Java practice is to be aware of default initialization values of member variables in Java and avoid redundant initializations.
Avoid Using Loop With Counter Variable
It is best to use the enhanced for loop instead of the old ‘For’ loop, as in some cases, the counter loop can become prone to errors. The counter variable can get altered on its own, disturbing the code at multiple points.’ Enhanced for’ loop is a good option in such a situation.
Finally, proper comments should be added to give an overview of your code and offer additional information that is not apparent in the code itself. Your code will be read by many people with varying levels of Java knowledge. Adding comments will make it readable for the tester, quality assurance engineer, maintenance staff, etc.
About the Creator
Programming School
𝑭𝒓𝒆𝒆 𝑶𝒏𝒍𝒊𝒏𝒆 𝑱𝒂𝒗𝒂 𝑷𝒓𝒐𝒈𝒓𝒂𝒎𝒊𝒏𝒈
𝑷𝒓𝒂𝒄𝒕𝒊𝒄𝒆 𝑸𝒖𝒊𝒛 & 𝑸𝒖𝒆𝒔𝒕𝒊𝒐𝒏𝒔
𝑳𝒆𝒂𝒓𝒏 𝑻𝒐 𝑴𝒂𝒌𝒆 𝑬𝒂𝒔𝒚
𝑪𝒐𝒓𝒆 𝑱𝑨𝑽𝑨 | 𝑨𝑾𝑺 | 𝑷𝒓𝒂𝒄𝒕𝒊𝒄𝒆 𝑻𝒆𝒔𝒕
www.synergisticit.com/free-java-test
Comments
There are no comments for this story
Be the first to respond and start the conversation.