How to Make Your ReactJS App Load Faster with Lazy Loading?
Lazy Loading Technique in ReactJS Development
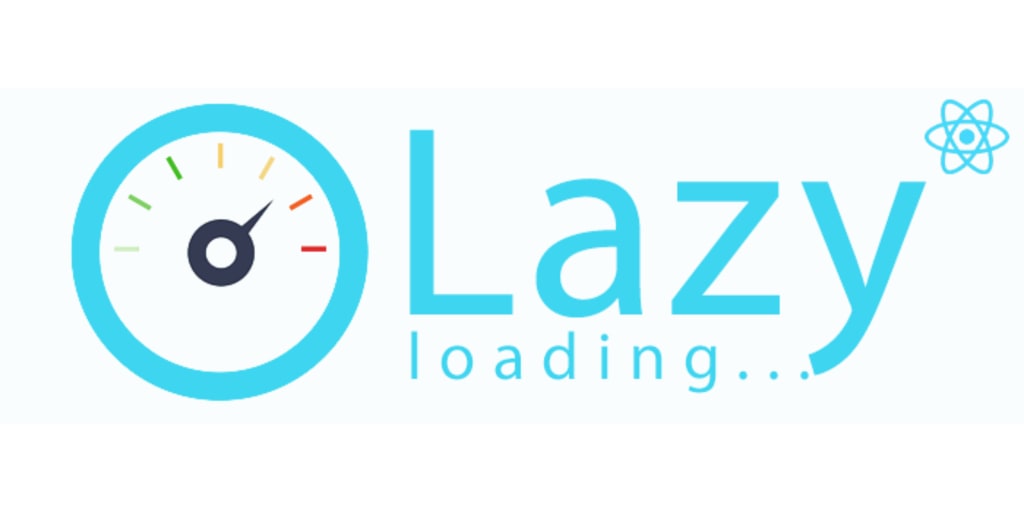
Lazy loading is a technique in ReactJS development that delays the loading of non-critical resources at page load time. This is done to improve the performance of a website or application by reducing the amount of data that needs to be transferred, reducing the loading time.
With lazy loading, components are only loaded when needed rather than being loaded all at once during the initial page load. This can lead to faster page load times and reduced memory usage, which can be especially beneficial for large applications or websites with many components.
Importance of Lazy Loading in ReactJS Development
Lazy loading can be implemented in ReactJS using the lazy function from the React library and the Suspense component. The lazy function allows you to define a component that can be loaded asynchronously. The Suspense component allows you to specify a fallback component to render while the lazy component is being loaded.
With these tools, you can ensure that your application or website only loads the components critical for the initial page load and delays the loading of non-critical components until they are needed. This can improve the overall performance of your application and provide a better user experience.
Implementing Lazy Loading Using React Suspense
Here's how you can implement lazy loading using React Suspense in a ReactJS application:
Use the lazy function from the react library to load components only when needed. The lazy function takes a function that returns a dynamic import statement, which tells React to load the module asynchronously when it is needed.

Wrap the lazy-loaded component inside a Suspense component, which allows you to specify a fallback component to render while the lazy component is being loaded.

If you have dependencies that the lazy component requires, you should also similarly lazy-load them.
Here is an example of a complete implementation of lazy loading in a ReactJS application:
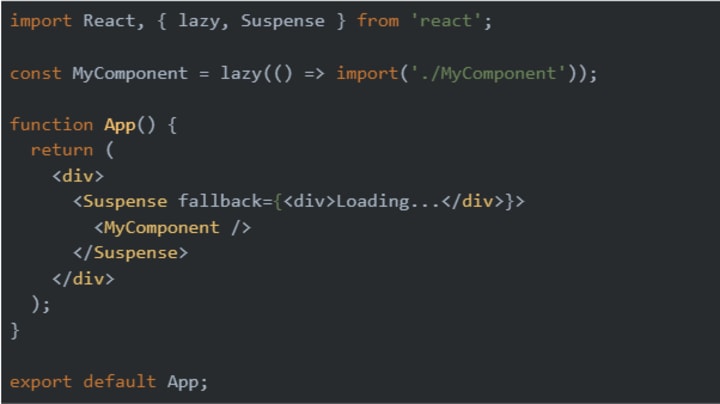
By using React Suspense for lazy loading, you can ensure that your components are only loaded when they are needed, which can improve the overall performance of your ReactJS application and provide a better user experience.
Implementing Lazy Loading Without Using React Suspense
While React Suspense is a convenient and recommended way to implement lazy loading in ReactJS, it is also possible to implement lazy loading without using it. Here's an example of how you could implement lazy loading using the useState and useEffect hooks:
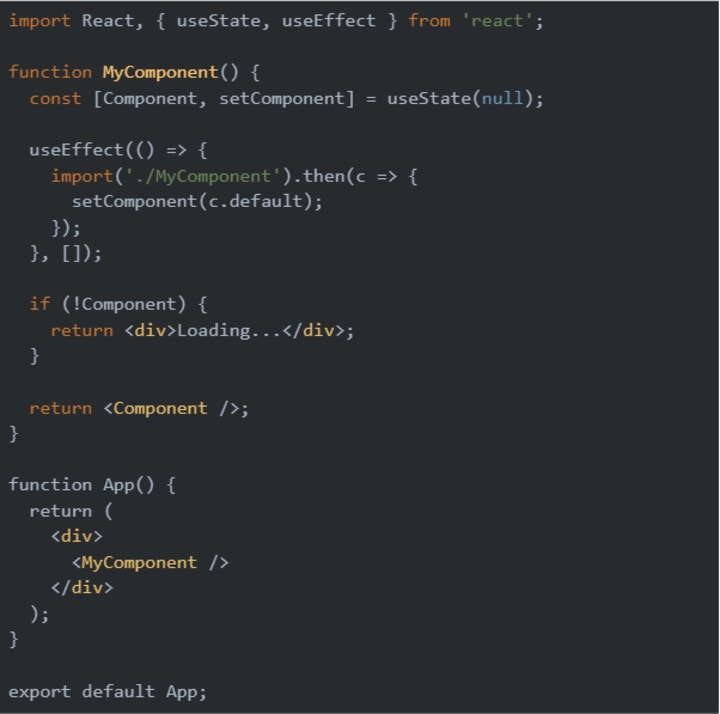
In this example, the MyComponent component is loaded dynamically using the import statement inside a useEffect hook. The hook sets the Component state to the lazy-loaded component once it has been imported, and until then, the component displays a "Loading..." message.
This approach to lazy loading can be helpful in cases where you want to implement lazy loading without using React Suspense, or if you want to have more control over the loading behaviour of your components. However, it requires a bit more code and logic to implement, so it may not be as convenient as React Suspense.
Conclusion
With these steps, you should now be able to implement lazy loading in your ReactJS application, which can help to improve its performance and the user experience. This helps to improve your application's performance and loading time, as well as reduce your users' data usage.
With React.lazy(), you can load components dynamically when needed instead of importing them all at once at the top level of your application. This helps reduce the amount of JavaScript that needs to be downloaded and parsed by the browser, making your application faster and more efficient.
About the Creator
Hardik Thakker
The CEO and Technical Writer of Albiorix Technology (www.albiorixtech.com) have over 12 years of experience in IT Operations, Branding and Marketing, Sales and Business Development, and Customer Relationship Management.
Comments
There are no comments for this story
Be the first to respond and start the conversation.