Top Java Collections Interview Questions (Advanced level Questionnaires)
A summary of the most frequently asked Collections questions in all IT Industry interviews.
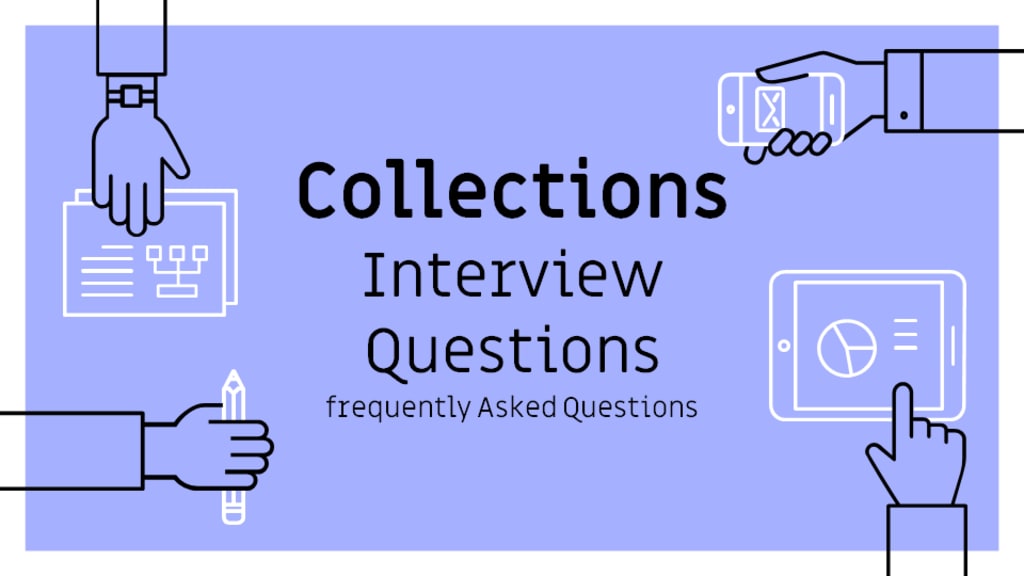
The questions given below are the most frequently asked question in IT Industries nowadays. Check out the summary of the most frequently asked questions categorized by Beginner, Intermediate, and advanced level in most of the IT industries. It doesn’t mean that these questions will actually be asked during your interview process, but yeah you can get a sense of what companies might ask you. If you want to add questions to this list — feel free to write in the comments/Response section.
Important: Please prepare all the questions so you can be confident when you need to answer any questions.
Java Collections Interview Question Categories…
- Beginner Level Questionnaires (0–2 years experience)
- Intermediate Level Questionnaires (1–3 years experience)
- Advanced level Questionnaires (3+ Experienced)

Advanced level Questionnaires (3+ Experienced)
1. How HashSet works internally in java?
A Java HashSet class represents a set of elements (objects).
- It does not guarantee the order of elements.
- It is achieved by storing elements as keys with the same value always.
- It constructs a collection that uses a hash table for storing elements.
- It contains unique elements.
- It inherits the AbstractSet class.
- It also implements the Set interface.
- It uses a technique to store elements is called hashing.
- HashSet uses HashMap internally in Java.
- [Important Iterator] HashSet does not have any method to retrieve the object from the HashSet. There is only a way to get objects from the HashSet via Iterator.
- HashSet has default initial capacity = 16.
- HashSet has default loadfactor = 0.75 or 75%
- The capacity may increase automatically when more elements to be store.
You can find the mechanism/ Internal working of HashSet here.
2. How HashMap Works Internally?
The reason behind adding this question again is to make sure you must be aware of it, Once you explain the HashSet — The interviewer may ask you about the internal working of HashMap.
3. What is the difference between Fail-Fast iterator and Fail-Safe iterator?
This is one of the most popular interview questions for the higher experienced java developers.
The main differences between Fail-fast and Fail-safe iterators are:
- Fail-fast throws ConcurrentModificationException while Fail-safe does not.
- Fail-fast does not clone the original collection list of objects while Fail-safe creates a copy of the original collection list of objects.
4. What is hash-collision in Hashtable? How it was handled in Java?
In Hashtable, if two different keys have the same hash value then it leads to hash-collision. A bucket of type LinkedList used to hold the different keys of the same hash value.
5. What is EnumSet in Java?
EnumSet is a specialized Set implementation for use with enum types. All of the elements in an enum set must come from a single enum type that is specified explicitly or implicitly when the set is created.
The iterator never throws ConcurrentModificationException and is weakly consistent.
#Advantage over HashSet:
All basic operations of EnumSet execute in constant time. It is most likely to be much faster than the HashSet counterparts.
It is a part of the Java Collections Framework since JDK 1.5.
6. How do you use a custom object as a key in Collection classes like HashMap?
- If one is using the custom object as a key then one needs to override the equals()and hashCode() method and one also need to fulfill the contract.
- If you want to store the custom object in the SortedCollections like SortedMap then one needs to make sure that equals() method is consistent with the compareTo() method.
- If inconsistent, then the collection will not follow their contracts, Because of that Sets may allow duplicate elements and the contract fails!
#Contract of hashCode() and equals() method
- If object1.equals(object2) , then object1.hashCode() == object2.hashCode() should always be true.
- If object1.hashCode() == object2.hashCode() is true does not guarantee object1.equals(object2)
7. What are concurrentCollectionClasses?
Since jdk1.5, Java Api developers had introduced a new package called java.util.concurrent that has thread-safe collection classes as they allow collections to be modified while iterating. The iterator is fail-safe that is it will not throw ConcurrentModificationException.
Some examples of concurrentCollectionClasses are:
1. ConcurrentHashMap
2. CopyOnWriteArrayList
8. How do you convert a given Collection to SynchronizedCollection?
One line code will work for you: Collections.synchronizedCollection(Collection collectionObj) — will convert a given collection to a synchronized collection.
9. How you can sort Arraylist objects?
One liner answer will work for you:
Collections.sort(yourList)
Or you can say the steps like,
Implement the Comparable interface for the given class
Now to compare the objects by a particular key, we will override the obj1.compareTo(obj2)
Then call method Collections.sort(yourList)
10. How to sort ArrayList in descending order?
Collections.sort(arraylist, Collections.reverseOrder());
Bonus Question: What are common algorithms used in the Collections Framework?
- Common algorithms used for searching and sorting. For example, a red-black algorithm is used in the sorting of elements in TreeMap.
- Most of the algorithms are used for the List interface but few of them are applicable for all kinds of Collection.
11. What is UnsupportedOperationException?
This exception is thrown to indicate that the requested operation is not supported.
For example, If you call add() or remove() method on the readOnly collection. We know readOnly collection can not be modified. Hence, UnsupportedOperationException will be thrown.
12. How to make Collections readOnly?
We can make the Collection readOnly by using the following lines code:
Collections.unmodifiableCollection(Collection c)
Collections.unmodifiableMap(Map m)
Collections.unmodifiableList(List l)
Collections.unmodifiableSet(Set s)
13. Why is String immutable or Final in Java?
There are many factors to make it final and Immutable in java.
- String Constant Pool: If the String is mutable, changing the string with one reference will lead to the wrong value for the other references.
- Synchronization and concurrency: making String immutable automatically makes them thread-safe thereby solving the synchronization issues.
- Class Loading: String is used as arguments for class loading. If mutable, it could result in the wrong class being loaded (because mutable objects change their state).
- Security: parameters are typically represented as String in network connections, database connection URLs, usernames/passwords, etc. If it were mutable, these parameters could be easily changed.
- Caching: when the compiler optimizes your String objects, it seems that if two objects have the same value (a=’Rax’, and b=’Rax’) and thus you need only one string object (for both a and b, these two will point to the same object).
14. Why char array is preferred to store passwords than String in Java?
String is immutable and it goes to the string pool. Once written, it cannot be overwritten. That means once you’ve created the String if another process can dump memory, there’s no way (aside from reflection) you can get rid of the data before garbage collection kicks in.
This is a security concern — but even using char[] only reduces the window of opportunity for an attacker, and it's only for this specific type of attack.
With plain String you have much higher chances of accidentally printing the password to logs, monitors, or some other insecure place. char[] is less vulnerable.
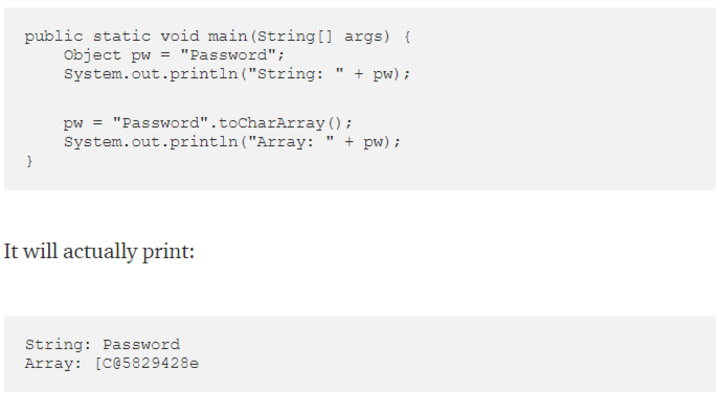
Because of the security concern it is better to store password as a character array.
15. Why wait and notify is declared in Object class instead of Thread?
Wait and notify is not just normal methods or synchronization utility, more than that they are communication mechanisms between two threads in Java. And Object class is the correct place to make them available for every object if this mechanism is not available via any java keyword like synchronized.
Remember synchronized and wait to notify are two different areas and don’t confuse that they are the same or related. Synchronized is to provide mutual exclusion and ensuring thread safety of Java class like race condition while wait and notify are communication mechanisms between two threads.
Locks are made available per Object basis, which is another reason wait and notify is declared in Object class rather than Thread class.
16. Can you override the static method in Java? if I create the same method in the subclass is it a compile-time error?
No, you can not override static method in Java but it’s not a compile-time error to declare the exact same method in a subclass, That is called method hiding in Java. We can only hide the static method in Java.
17. Why do you think we need Java collections? (Rarely asked)
The Java collection framework provides the developers to access prepackaged data structures as well as algorithms to manipulate data.
18. Check if Brackets are paired or not in the given expression using Stack / Deque.
You can find the full explained answer here.
You May Also Like,
Java Collections - Beginner Questionnaire
Java Collections - Intermediate Questionnaire

Get my stories in your feeds by subscribing to me, or become a vocal+ member to read all stories of thousands of other writers, participate in all challenges and get a payout with low fees and less payout threshold on Vocal Media.
© Originally Published on Top Collections — Java Interview Questions, also republished on Medium by Author Rakshit Shah (Me).
About the Creator
Rakshit Shah
I am Computer Engineer and love to make websites and software. I am really eager to know about anything. I am curious to read and write cool stuff.
Comments
There are no comments for this story
Be the first to respond and start the conversation.