Scala Beginner Series (1) : Basics
This article will cover the fundamentals of Scala language.
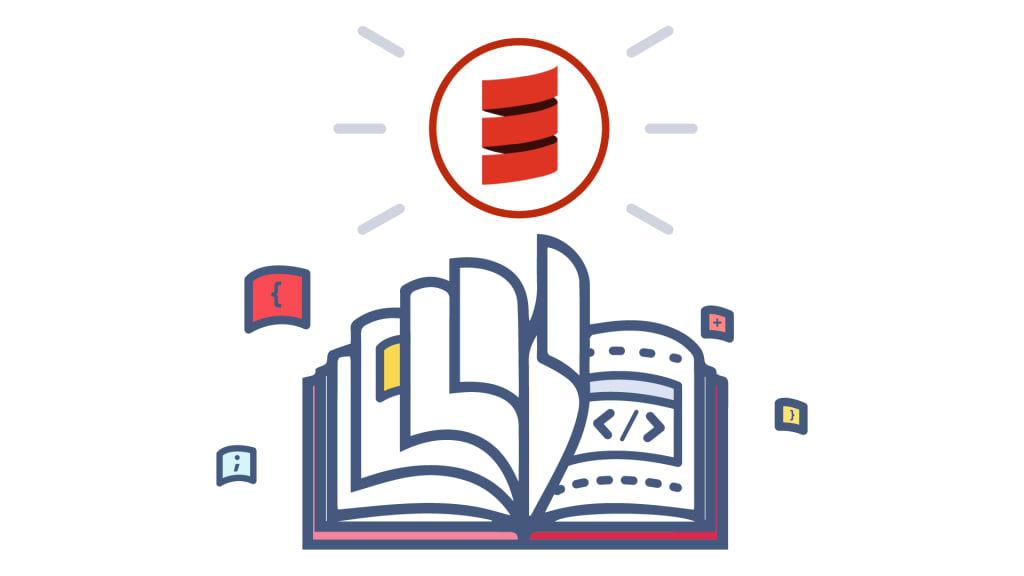
This series is all about the taste of Scala. It is best suitable for all the newbies in Scala. In this article we will cover the fundamentals of Scala language.
Values
In Scala, we work with values:
Values are used to define constants. val modifier means constant or immutable that means we cannot change its value once it is created. Thus reassignment to val is prohibited.
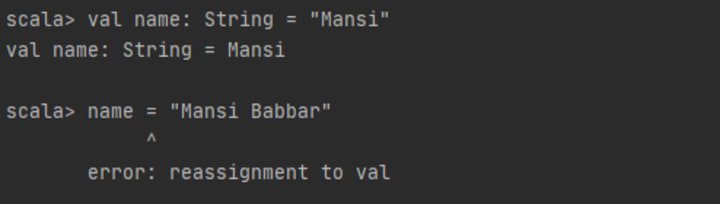
It is evaluated at time of definition only. Once evaluated, it reuses same value for all references of it.
Variables
Scala also allows us to define mutable values. Variables are used to define mutable reference to a value. var modifier means changeable or mutable that means we can change its value throughout the program lifetime . Thus reassignment to var is allowed.
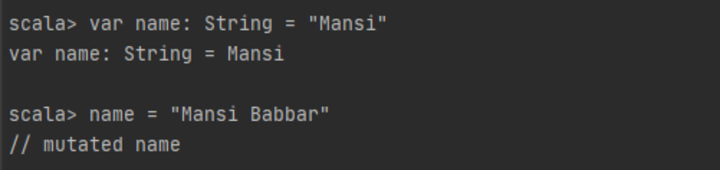
We do have the notion of a variable in Scala, but it’s heavily discouraged. In general, we work with immutable data structures: any intended modification to an existing instance should return a new (modified) instance.
Types
In Scala, we don’t always need to specify the data type of our value, because the compiler is smart enough to infer that for us. We can also write:

We can see, the compiler automatically inferred the data type of the value.
Strings
Strings in Scala are similar to what we see in other languages, but with some special functionalities:
Strings are defined as:

Whenever compiler encounters a string literal in the code, it creates a String object of java.lang.String class with its value.
Methods used to obtain information about an object are known as accessor methods.
One accessor method that can be used with strings is the length() method, which returns the number of characters contained in the string object.
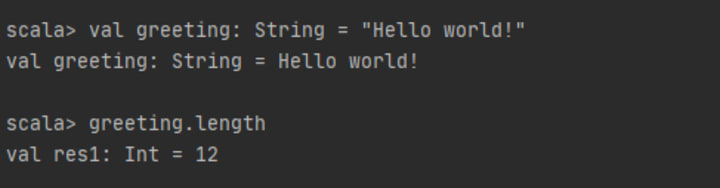
The String class includes a method concat for concatenating two strings. But strings are more commonly concatenated with the + operator.
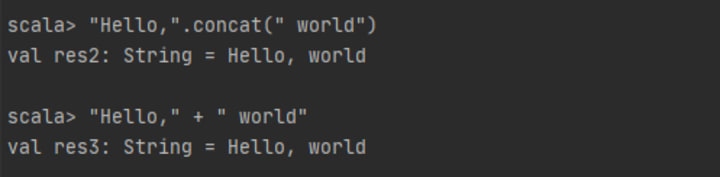
String interpolation can also be done using s string interpolator. It allows the direct usage of variable in processing a string, by injecting a value with the $ sign.
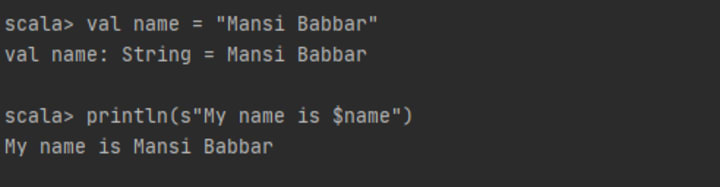
Expressions
In Scala, we work with values and we compose them to obtain other values. The composition structures are expressions, and they’re exactly what we expect.
Previously we have defined values assigned to literals. However, it is more accurate to say that they are assigned to the return value of expressions.
Thus expression is a single unit of code that that can be reduced to a value. It can be a literal, a calculation, or a function call. An expression has its own scope, and may contain values local to the expression block.
We can define values based on expressions as:

In Scala, we can also define expression blocks. Multiple expressions can be combined using curly braces to create a single expression block. The last expression in the block is the return value for the entire block.

If-structures are expressions as well.
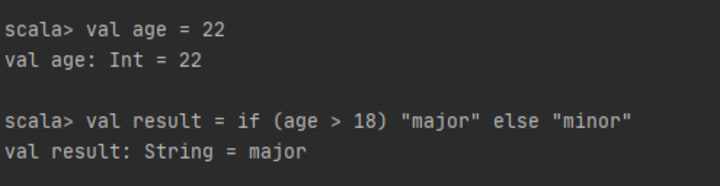
In C-like languages, we have equivalent ternary operator, but in Scala, it’s much more readable because we can chain if-expressions in endless if/else structures without the risk of misunderstanding any logic.
We have other type of expressions as well, like for-expressions, match-expressions, etc. We’ll talk about them later.
Functions
Scala has both functions and methods and we use the terms method and function interchangeably with a minor difference. A Scala method is a part of a class which has a name and a signature, where as a function in Scala is a complete object which can be assigned to a variable.
Functions are declared and defined as:
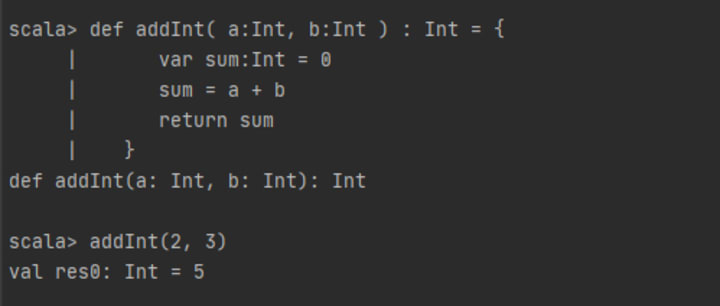
So we have:
- def
- function name
- arguments in the form of arg : Type
- : ReturnType
- =
- then a single expression the function will return
Recursion plays a big role in pure functional programming and Scala supports recursive functions very well. In Scala, we don’t think in terms of loops. We think in terms of recursion.
Recursion means a function can call itself repeatedly.
A simple example of recursion is demonstrated as:

It is mandatory to mention the return type of recursive functions.
The Unit Type
Finally, I’ll wrap this article with the type of expressions that don’t return any meaningful value, i.e. Unit. It is the equivalent of void functions in other languages. It is used when nothing needs to be returned by the function.
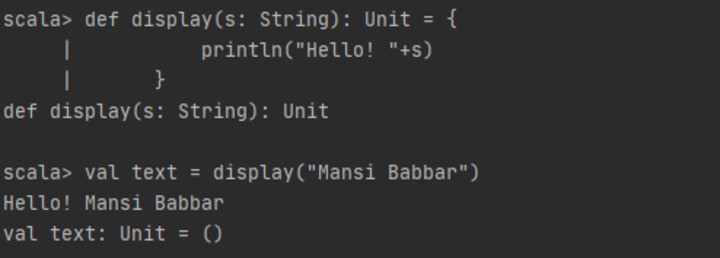
An example is the println() function, which returns Unit. It is a type containing a single value denoted ().
Expressions returning Unit are called side effects, because they have nothing to do with computing meaningful values. In pure functional programming, we tend to keep side effects to a minimum.
Stay Tuned…
Stay tuned for our next part of Scala Beginner Series where we will cover the object oriented nature of Scala. You don’t want to miss it!
Originally published on Medium by Mansi Babbar.
Comments
There are no comments for this story
Be the first to respond and start the conversation.