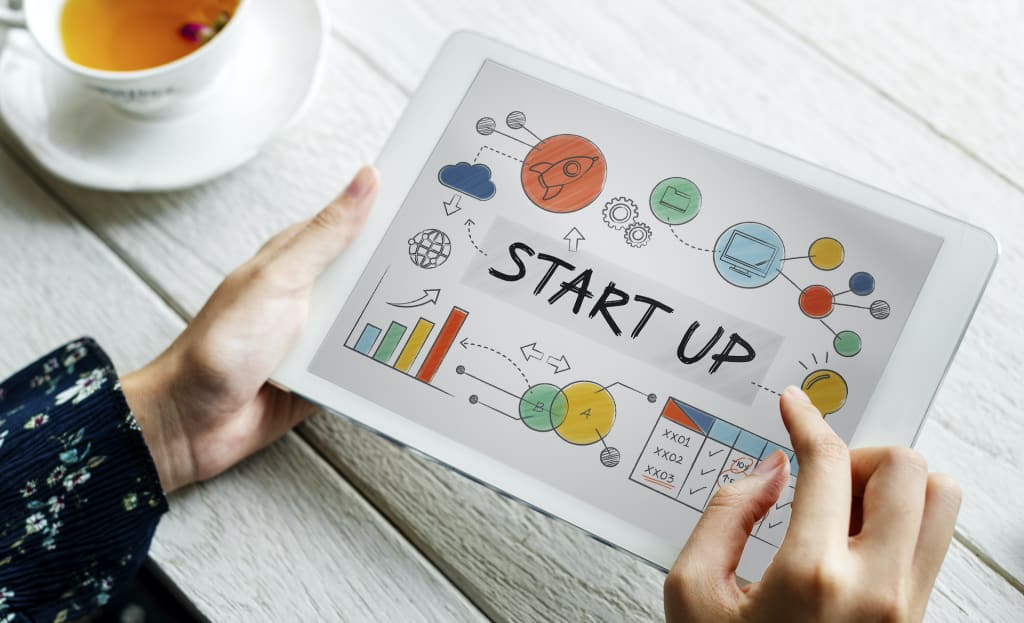
Hello all, we are back with our next article. In case you would like to refer our previous articles, you can find them here.
In our last article we discussed about MVC architecture, its problem and pros and cons.
The major problems that comes with MVC architecture in iOS development are
- UI and logic gets tightly coupled as both are present in single class UIViewController.
- Tight coupling between UI and logic violates Single Responsibility Principle
- As logic cannot be separated from UI code, unit testing also becomes very difficult.
The above problems are very well addressed by modern front end development architectures and one of them is MVP architecture.
Let us to understand this architecture in detail.
What is MVP?

As show in above diagram. MVP distributes application code in 3 components or layers.
- View: This component is also known as Presentation layer. In iOS development any class which is subclass of UIViewController, UIView or any UI component, comes under presentation layer. This component is what it is visible to user. This component is usually dumb and does not contains any decision making and is driven by Presenter component
- Presenter: This component is also known as Business layer and classes under this component are responsible to contain logic part. Presenter class is generally a plain swift class or NSObject subclass if using Objective C. As this component contains the logic, all the decision making takes place in Presenter component and this component tells the View component what to do on each user action. For example whether to show success/error message or navigate user to other screen.
- Model: This component is also known as Data layer and classes under this component are responsible to contain data models and domain entities that generally represents raw data. For example, a User class representing application user. Model class is generally a plain swift class or NSObject subclass if using Objective C.
So it is clear from above explanation that MVP pattern divides UI and logic code in View and Presenter components respectively. By doing this separation MVP follows Single Responsibility principle and code unit testing becomes very easy.
Communication between View and Presenter
An important point to not here is that how communication between View and Presenter classes takes place.
View holds the strong reference to Presenter component to pass all the user actions and Presenter holds a weak reference of View component to tell the view what to do on each action.
Example
Let us now see how we can implement MVP architecture using an example. We will use swift programming language for this example.
We will take a very simple example of login screen which is almost part of every business application.
Use case
Consider a login screen with 2 fields email and password and a login button. When user presses the login button, email and password entered by the user should be validated and user should be authenticated and taken to home screen on successful authentication.
Validation rules should be as below
- Email should not be blank
- Password should not be blank
- Password should contain at least 8 characters.
Let us see each component or class in detail.
View component
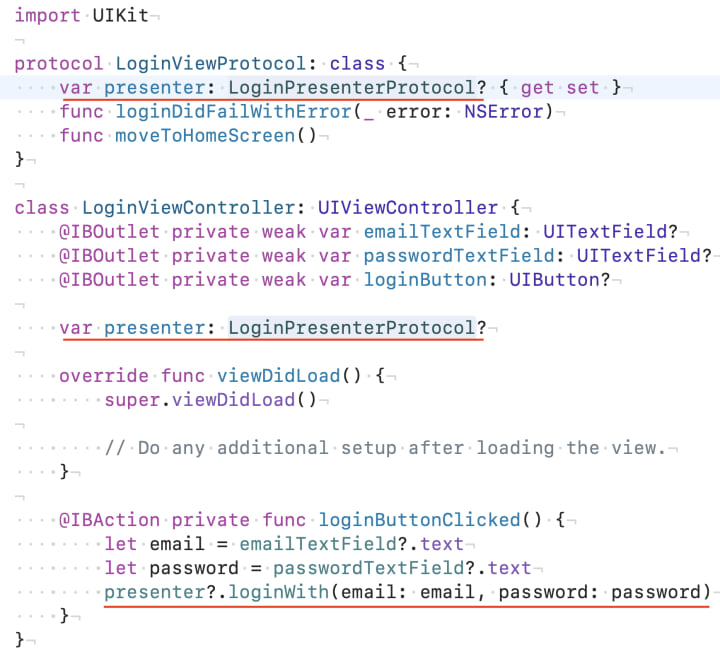
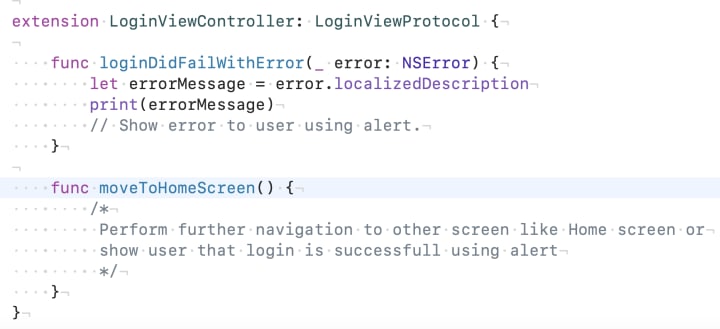
We can conclude below points from above pictures
- LoginViewController which is part of View component is subclass of UIViewController.
- It contains strong reference to Presenter component via LoginPresenterProtocol.
- It listens to updates provided by Presenter class via LoginViewProtocol.
- When user clicks login button, View component notifies Presenter component that user has pressed login button and passes email and password entered by user, through that event.
Presenter component
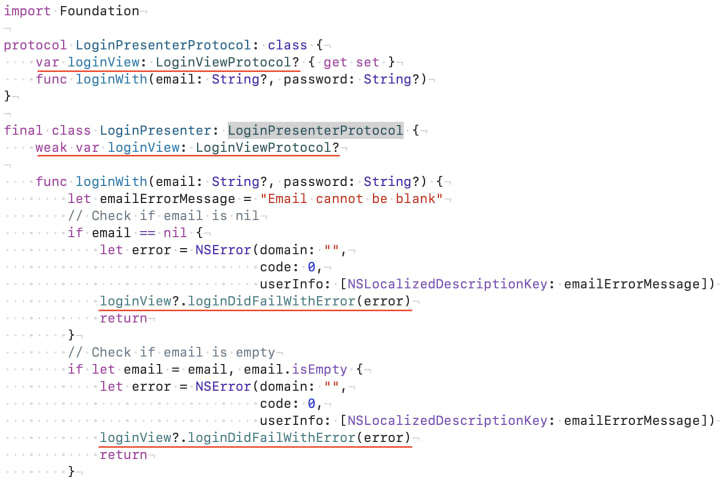
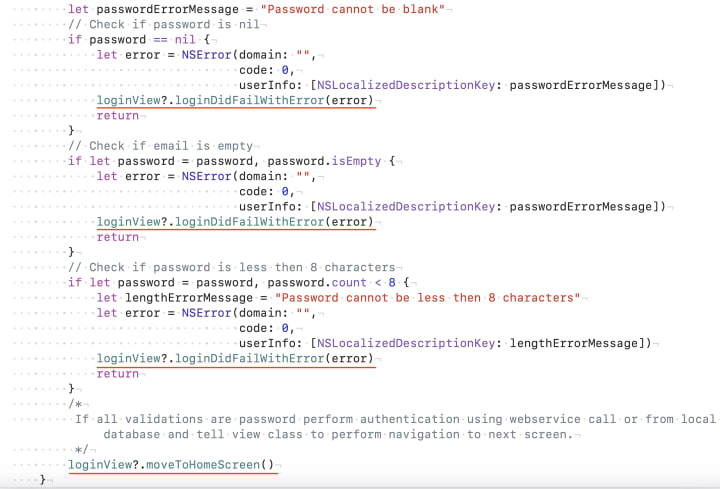
We can conclude below points from above pictures
- LoginPresenter is plan swift class that conforms to LoginPresenterProtocol.
- This class contains weak reference of View component via LoginViewProtocol
- When Presenter gets information from View component, it validates email and password against each business rules that we defined above while defining the example.
- For each failed validation Presenter component tells View component, what error message should be shown to user.
- Once all validations are passed Presenter component tells View component to navigate user to home screen.
Model Component
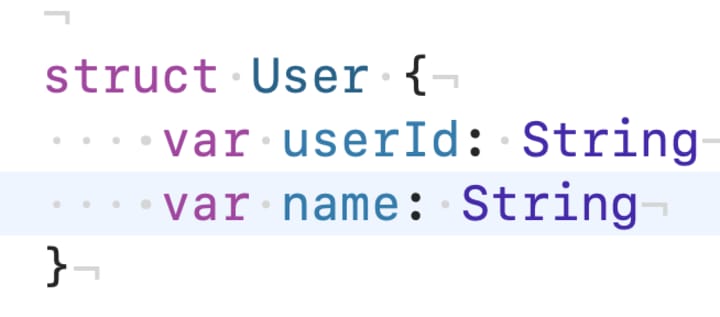
This component is very simple it represents the real-world user that logs into the application. It contains 2 properties for now, but we can add other required properties as well. This can be a plan swift class. However in iOS it is best practice to use struct instead of class for data model.
With this we have come to an end of our article. Hope we were able to explain what MVP architecture is, how it addresses problems of MVC architecture and how can we implement this in iOS.
We have discussed about MVP and its basic concepts in this article. We will highlight some more concepts of MVP architecture and we will see how those concepts will help in unit testing in our next article.
Thanks for reading this article. If you have any queries related to this topic or iOS, Objective C and Swift. Please write us at [email protected] or direct message us on instagram at conficle(instagram username).
Also keep watching this space for upcoming articles on iOS development, software development and technology concepts.
Comments
There are no comments for this story
Be the first to respond and start the conversation.